When editing boolean values in MVC the default option is to use a checkbox. This results in the following Create page when using the default scaffolding
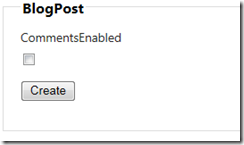
This is fine and works OK but sometimes you want something more..’fancy’. ASP.NET MVC ships with Jquery.UI so why no use the slider of JQuery.UI to create a nice toggle switch?
In this post I will use and modify a JQuery.UI plugin that will result in the following. The plugin is based on http://taitems.github.io/UX-Lab/ToggleSwitch/ which had some errors in my opinion.
There are a two parts to this:
- Create a HTMLHelper extension method that renders a <select> element for the boolean property with <option> elements for true and false
- Change the rendered <select> element to a jquery slider using a JQuery.UI plugin
Creating the HTMLHelper
public static class HtmlHelperExtensions
{
public static MvcHtmlString ToggleSwitchFor<TModel>(this HtmlHelper<TModel> htmlHelper, Expression<Func<TModel, bool>> expression)
{
return htmlHelper.DropDownListFor(expression, new[]
{
new SelectListItem { Text = "No", Value = "false" },
new SelectListItem { Text = "Yes", Value = "true" }
}, new { @class = "toggleswitch" });
}
}
This adds a HTMLHelper method that renders a dropdown list which comes down to a single select HTML element. There are two options in the select : one for true and one for false. To use it we just do
@Html.ToggleSwitchFor(model => model.CommentsEnabled)
And we get the following html
<select class="toggleswitch" data-val="true" data-val-required="The CommentsEnabled field is required." id="CommentsEnabled" name="CommentsEnabled"><option value="false">No</option><option value="true">Yes</option></select>
This is all what we need to get our JQuery plugin going.
Creating the JQuery plugin
The plugin uses the JQuery.UI slider to create a toggle switch. This means that any styling or theme you might have for the slider will work for the toggle as well. The plugin transforms the select element into two labels with a slider in between. When you click on a label or move the slider the select control will be updated as well and when you do form post the values get updated. The http://taitems.github.io/UX-Lab/ToggleSwitch/ had two errors when I tried it
- The change event was just passing the change event of the slider. This meant even if the boolean property did not change (you moved the slider a little) you would still get a change event. In this version the change event is only fired when the value really changed.
- The plugin did not allow chaining.
- The normal ‘change’ event of JQuery was no longer fired. This is fixed by not having a change event on the control itself but just fire the existing change event.
To use it you need to add the CSS and JS of the plugin to your page. You also need the default JQuery UI js and css (which is not referenced by default!). Once you have done that you can activate the plugin for a control:
$(document).ready(function () {
$("#CommentsEnabled").toggleSwitch().change(function() {
alert("Changed!!");
});
});
I attached a sample MVC application as well as the JQuery.UI.ToggleSwitch plugin.
ToggleSampleMVC4.zip (771,1 KB)jquery.ui.toggleswitch.zip (1,73 KB)