1. juni 2013 19:30 by martijn in
.net, jQuery, MVC, typescript, Weld In this post I want to talk about a little tool called Weld I wrote to make integrating client-side TypeScript and server side MVC C# easy.
Imagine you have a server-side method that calculates some value you want to use client side. In this sample project I want to calculate the sum of two values I have in input fields.
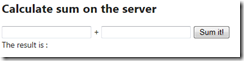
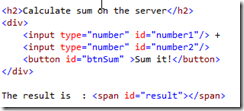
Server-side I have this action-method in my home controller.
public int Sum(int x,int y)
{
return x + y;
}
Now to access this method client I just need to add a Weld attribute. For this I first install Weld using NuGet.
Just “Install-Package Weld” in your NuGet package manager console. This will install Weld as well a TypeScript.Compile and JQuery typings into your project.
TypeScript.Compile auto compiles all your typescript files post-build. This enables you to detect any problems caused by server side changes.
The basic process :
- Normal compilation
- Weld generates TypeScript code for the classes your decorated with Weld attributes
- TypeScript compiles all files in your project with build actions 'TypeScriptCompile'
For this sample I add the AjaxMethod (Weld.Attributes.AjaxMethod) attribute to the ‘Sum’ action.
Compile the project. Weld now has generated a proxy class for my home controller. I just need to include it and used it in the project. It is located in the /Scripts/Weld folder.
I add the generated homecontroller.ts to my project. I my main TypeScript file I write the following to hook it all up:
/// <reference path="Weld/HomeController.ts" />/// <reference path="typings/jquery/jquery.d.ts" />
$(document).ready(function () {
$("#btnSum").click(function () {
HomeController.prototype.Sum($("#number1").val(), $("#number2").val(), (result) => {
$("#result").text(result);
});
});
});
As you can see the details of the Ajax communication are handled by weld and you get a nice interface to your server side API :)